/** * Simple scenario where a method receives and returns a modified test message. * @param msg Message String. * @return Returns the incoming message, prefixed with a greeting. */ public static String hello(String msg) { return "Hello there, you said: " + msg + "."; }
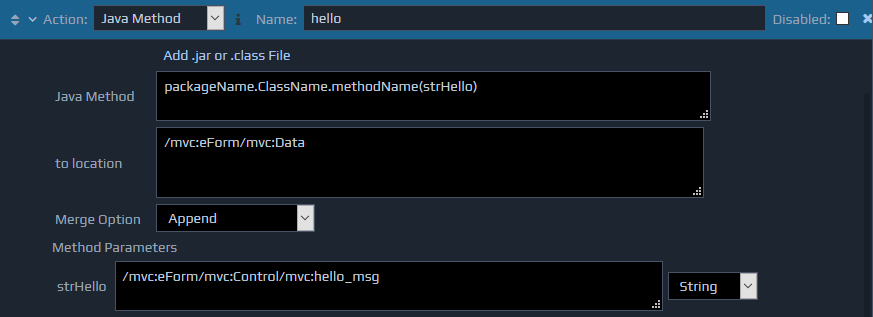
When you select the Java Method Action in one of your rules, you will be presented with a set of fields to complete, similar to all other Actions in WebMaker. For example:
Java Method - Fully qualified method name and signature. For example, com.hyfinity.xengine.effectors.JavaMethod.hello(msg), where the Package name is
Your FactBase in this instance might look like:
com.hyfinity.xengine.effectors, the Class name is
JavaMethodand the Method name is
hello. to location - Location in the FactBase where the return information will be placed. For example,
/mvc:eForm/mvc:Date/mvc:FormData/java_return. This can be any arbitrary location of your choice. Method Parameters - {A list of parameters required by the method signature}. For example,
msg. Assuming this is of type String, you can provide any valid XPath, such as 'Hi there Java Method' or provide an XPath such as
/mvc:eForm/mvc:Date/mvc:FormData/message_to_java.
<eForm xmlns="http://www.hyfinity.com/mvc" xmlns:mvc="http://www.hyfinity.com/mvc"> <Control> ... </Control> <Data> <formData> <message_to_java>Hi there Java Method</message_to_java> </formData> <java_return></java_return> </Data> </eForm>
Method Parameters
Once you have defined your method call and click out of the Java Method edit box, WebMaker will parse the method signature and present a list of defined parameters. For each parameter, you can use any valid XPath to bind your data that will be sent to the Java Method.
Data Types and mappings
For each parameter, you can indicate the matching WebMaker data type. The available types are the same as those available in the Page Designer under "Data Constraints|Data Type" and include:
Boolean - Mapped to Java primitive type
boolean. String - Mapped to
java.lang.String. Number - Mapped to
int,
short,
long,
floator
double. The Number data type therefore acts a more general wrapper for the underlying Java types. This means that a method call such as myMethod(num), where num is of type Number, could match myMethod(int myInteger), myMethod(float myFloatingPointNum), etc. Date - Mapped to
java.util.Date. Additionally, dates will be automatically converted between
XML Schema Dateand
Java Dateformats. XML - Mapped to
org.w3c.dom.Node. Maps any XML fragment from the
FactBaseto a
Nodeobject.
Handling Return Information
WebMaker will automatically determine the return type and attempt to perform the following mappings:
Node - Maps any XML Node, including Document, Element, etc. and inserts it into the FactBase
to locationas an XML fragment. String - Sets the text of the
to locationto the returned String value. Date - Converts the Java Date to an XML Schema Date and sets the text of the
to locationto the returned Date value. Other types - For all other return types WebMaker will perform a toString() operation on the return type and set the text of the
to locationto the returned string value, if a value is produced.
Compiling and deploying the Java classes
The .class or .jar files must be in the Java class-path to enable WebMaker to use them. Typically, these files will be located in {WebMaker install location}/.../tomcat-runtime/webapps/{your-app-name}/WEB-INF/classes or {WebMaker install location}/.../tomcat-runtime/webapps/{your-app-name}/WEB-INF/lib respectively. Note: It is recommended that JAR files are generally used for simplicity and for portability.
Wrapping native Java components
Please note that method definitions must be public and static to enable invocation from WebMaker. If you wish to utilise instance methods or methods that accept or return more complex types then you can write your own method that is public and static and handles one of the recognised WebMaker parameter and return types. Your method can then perform the invocation of the arbitrary Java components as required.
Examples
For more detailed examples of Java Method invocations, including methods that wrap other standard Java methods, please search the WebMaker Forum for Barcode,
XML Digital Signaturesand
XML Encryption and Decryption. Troubleshooting
Debugger Trace and Exceptions - Common with other WebMaker actions, the Java Method Action will produce various logs depending on your log settings. You can view these in the Debugger message logs. The logs will identify issues during the parsing stage of your method call and also any resulting issues that arise during the actual invocation of the method. WebMaker will attempt to catch any Exceptions thrown by the underlying methods and wrap them into the Debugger message trace during invocation.
Statement Parsing - Likely error messages during the parse stage will be due to incorrect names for the Package, Class or Method. The parameters must be of the correct types and in the right order, otherwise WebMaker will not find a match for the method. You will also encounter issues if the method is not declared as public and static.